Tcl/Tk
Tcl/Tk は、widget と呼ばれる部品を使って簡単に GUI アプリケーションを作成できますがTkinterはこれを Python で使えるようにしたものです
次のサイトを見ると概要が分かります
M.Hiroi's Home Page / Tcl/Tk お気楽 GUI プログラミング入門 (nct9.ne.jp)
Tk Commands, version 8.6.14 (tcl-lang.org)
デジタル時計の例です
proc show_t {} {
global buffer
set buffer [clock format [clock seconds] -format "%H:%M:%S"]
after 1000 show_t
}
label .l0 -textvariable buffer
pack .l0
show_t
wish86t.exe show_t.tcl
Tkinterのデジタル時計
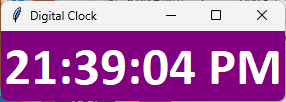
import tkinter as tk
from time import strftime
def time():
# 現在の時刻を取得
string = strftime('%H:%M:%S %p')
# 時刻をラベルに更新
label.config(text=string)
# 1秒ごとにtime関数を呼び出す
label.after(1000, time)
# Tkinterウィンドウの作成
root = tk.Tk()
root.title("Digital Clock")
# ラベルの作成
label = tk.Label(root, font=('calibri', 40, 'bold'), background='purple', foreground='white')
# 時計の位置を設定
label.pack(anchor='center')
# 時計を開始
time()
# ウィンドウを表示
root.mainloop()
Tkinterとttk
Tkinterよりttkの方が綺麗で使いやすくなっています。比較したプログラムを掲載します
おまけで他のウィジェットも追加してます
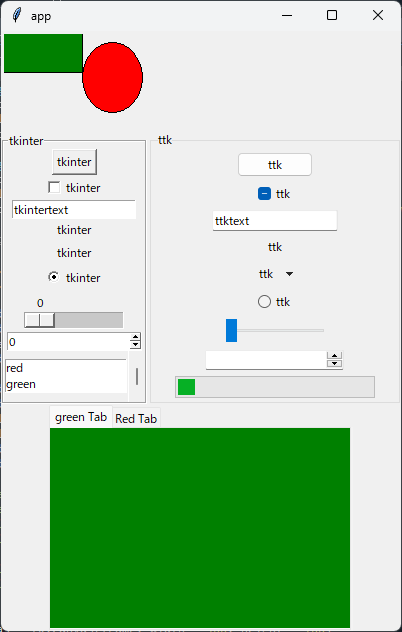
import tkinter as tk
from tkinter import ttk
def get_text():
text1 = text_1.get()
label.config(text=text1)
canvas.delete("oval")
def get_text_ttk():
text2 = text_2.get()
label_ttk.config(text=text2)
canvas.create_oval(140, 10, 80, 80, fill = 'red', tag="oval")
root = tk.Tk()
root.title("app")
root.geometry("400x600")
paned_window2 = tk.PanedWindow(root, height=100)
paned_window2.pack(expand=True, fill='both')
frame = tk.Frame(root)
paned_window2.add(frame)
paned_window = tk.PanedWindow(root)
paned_window.pack(expand=True, fill='both')
labelframe = tk.LabelFrame(root, text="tkinter")
labelframe_ttk = ttk.LabelFrame(root, text="ttk")
paned_window.add(labelframe)
paned_window.add(labelframe_ttk)
'''
frame = tk.Frame(root)
frame.pack(padx=20,pady=10)
labelframe = tk.LabelFrame(root, text="tkinter")
labelframe.pack(padx=20, pady=20, side="right")
labelframe_ttk = ttk.LabelFrame(root, text="ttk")
labelframe_ttk.pack(padx=20, pady=20, side="left")
'''
canvas = tk.Canvas(frame, width = 400, height = 100)
canvas.create_rectangle(0, 0, 80, 40, fill = 'green')
canvas.create_oval(140, 10, 80, 80, fill = 'red', tag="oval")
canvas.pack(fill="y")
#
button = tk.Button(labelframe, text="tkinter", command=get_text)
button_ttk = ttk.Button(labelframe_ttk, text="ttk", command=get_text_ttk)
button.pack(expand=True)
button_ttk.pack(expand=True)
#
checkbutton = tk.Checkbutton(labelframe, text="tkinter")
checkbutton_ttk = ttk.Checkbutton(labelframe_ttk, text="ttk")
checkbutton.pack(expand=True)
checkbutton_ttk.pack(expand=True)
#
text_1 = tk.StringVar()
text_2 = tk.StringVar()
text_1.set("tkintertext")
text_2.set("ttktext")
entry = tk.Entry(labelframe, text="tkinter",textvariable=text_1)
entry_ttk = ttk.Entry(labelframe_ttk, text="ttk",textvariable=text_2)
entry.pack(expand=True)
entry_ttk.pack(expand=True)
#
label = tk.Label(labelframe, text="tkinter")
label_ttk = ttk.Label(labelframe_ttk, text="ttk")
label.pack(expand=True)
label_ttk.pack(expand=True)
#
menubutton = tk.Menubutton(labelframe, text="tkinter")
menubutton_ttk = ttk.Menubutton(labelframe_ttk, text="ttk")
menu = tk.Menu(menubutton, tearoff=False)
menu.add_command(label="Option 1")
menu.add_command(label="Option 2")
menubutton.config(menu=menu)
menubutton_ttk.config(menu=menu)
menubutton.pack(expand=True)
menubutton_ttk.pack(expand=True)
#
radio = tk.Radiobutton(labelframe, text="tkinter")
radio_ttk = ttk.Radiobutton(labelframe_ttk, text="ttk")
radio.pack(expand=True)
radio_ttk.pack(expand=True)
#
scale = tk.Scale(labelframe,orient="horizontal")
scale_ttk = ttk.Scale(labelframe_ttk)
scale.pack(expand=True)
scale_ttk.pack(expand=True)
#
radio = tk.Spinbox(labelframe, increment=1, from_=0, to=5)
radio_ttk = ttk.Spinbox(labelframe_ttk, increment=1, from_=0, to=5)
radio.pack(expand=True)
radio_ttk.pack(expand=True)
listvar=tk.StringVar()
listvar.set(['red','green','white'])
listbox=tk.Listbox(labelframe, height=2, width=20, listvariable=listvar, selectmode='multiple')
scrollbar = tk.Scrollbar(labelframe, orient=tk.VERTICAL, command=listbox.yview)
listbox["yscrollcommand"] = scrollbar.set
scrollbar.pack(side="right")
listbox.pack(side="left",expand=True)
progressbar_ttk = ttk.Progressbar(labelframe_ttk, length=200)
progressbar_ttk.pack(expand=True)
progressbar_ttk.start(10)
ttknotebook=ttk.Notebook(root,height=300,width=300)
frame1=tk.Frame(root,background='green')
frame2=tk.Frame(root,background='red')
ttknotebook.add(frame1,text='green Tab')
ttknotebook.add(frame2,text='Red Tab', padding=5)
ttknotebook.pack(expand=True)
root.mainloop()
サンプルが掲載されているサイト
https://ultrapythonic.com/category/tkinter/
widget
bell
bind
bindtags
bitmap
busy
button
canvas
checkbutton
clipboard
colors
console
cursors
destroy
entry
event
focus
font
fontchooser
frame
geometry
grab
grid
image
keysyms
label
labelframe
listbox
lower
menu
menubutton
message
option
options
pack
panedwindow
photo
place
radiobutton
raise
safe::loadTk
scale
scrollbar
selection
send
spinbox
text
tk
tk::mac
tk_bisque
tk_chooseColor
tk_chooseDirectory
tk_dialog
tk_focusFollowsMouse
tk_focusNext
tk_focusPrev
tk_getOpenFile
tk_getSaveFile
tk_library
tk_menuSetFocus
tk_messageBox
tk_optionMenu
tk_patchLevel
tk_popup
tk_setPalette
tk_strictMotif
tk_textCopy
tk_textCut
tk_textPaste
tk_version
tkerror
tkwait
toplevel
ttk::button
ttk::checkbutton
ttk::combobox
ttk::entry
ttk::frame
ttk::intro
ttk::label
ttk::labelframe
ttk::menubutton
ttk::notebook
ttk::panedwindow
ttk::progressbar
ttk::radiobutton
ttk::scale
ttk::scrollbar
ttk::separator
ttk::sizegrip
ttk::spinbox
ttk::style
ttk::treeview
ttk::widget
ttk_image
ttk_vsapi
winfo
wm